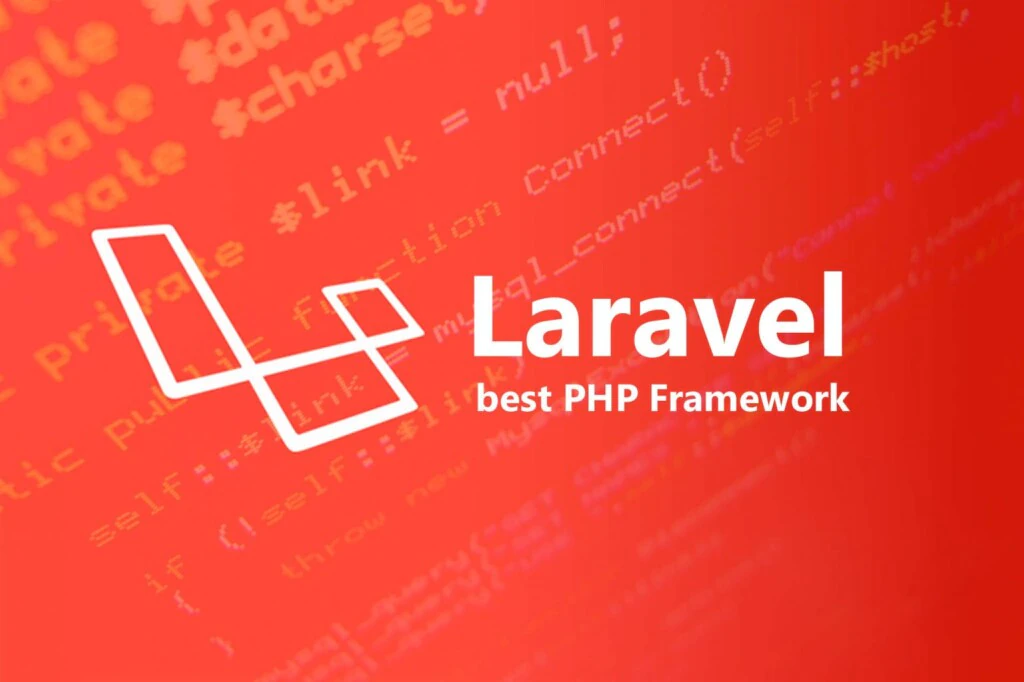
In modern web applications, handling time-consuming tasks synchronously can significantly slow down your application and degrade the user experience. Laravel workers offer a powerful solution by offloading these tasks to background processes, ensuring your application remains responsive and efficient.
In this comprehensive guide, we'll walk you through setting up a Laravel worker server on Ubuntu. We'll cover everything from understanding what Laravel workers are to configuring and supervising them in a production environment.
1. Understanding Laravel Workers
What Are Laravel Workers?
Laravel workers are background processes that handle tasks outside of the normal request-response cycle. They listen to a queue for new jobs and process them asynchronously. This is especially useful for tasks that take a long time to execute, such as sending emails, processing images, or interacting with external APIs.
Common Use Cases
- Email Sending: Offload email sending to the queue to avoid slowing down user interactions.
- Notifications: Send push notifications or SMS messages in the background.
- Data Processing: Handle intensive data operations without affecting the application's performance.
- Third-Party API Calls: Interact with external services without making the user wait.
2. Prerequisites
Before we begin, ensure you have the following:
System Requirements
- Ubuntu 20.04 LTS or later.
- PHP 8.0 or newer.
- Composer for dependency management.
- Laravel 9 or newer installed.
- Database (MySQL, PostgreSQL, etc.) configured.
Environment Setup
Ensure your Ubuntu server has all the necessary packages:
sudo apt update sudo apt install php-cli php-mbstring php-xml php-zip php-mysql unzip
Install Composer globally:
curl -sS https://getcomposer.org/installer | php sudo mv composer.phar /usr/local/bin/composer
3. Setting Up Queue Configuration
Laravel supports several queue drivers. For this guide, we'll use Redis for its performance and reliability.
Installing and Configuring Redis
Install Redis on Ubuntu:
sudo apt install redis-server
Enable Redis to start on boot and start the service:
sudo systemctl enable redis-server.service sudo systemctl start redis-server.service
Install the Redis PHP extension:
sudo apt install php-redis
Restart PHP-FPM or Apache:
sudo systemctl restart php7.4-fpm.service # or for Apache sudo systemctl restart apache2.service
Configuring .env
File
Update your Laravel application's .env
file to use Redis:
QUEUE_CONNECTION=redis
Ensure Redis settings are correct in config/database.php
and config/queue.php
.
4. Creating and Dispatching Jobs
Generating a Job Class
Use Artisan to create a new job:
php artisan make:job ProcessData
This command creates a new job class in app/Jobs/ProcessData.php
.
Implementing the Job Logic
Edit the handle
method in the job class:
namespace App\Jobs; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; class ProcessData implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable; public function handle() { // Your job logic here } }
Dispatching Jobs to the Queue
In your controller or service class:
use App\Jobs\ProcessData; class DataController extends Controller { public function process() { // Dispatch the job ProcessData::dispatch(); return response()->json(['status' => 'Job dispatched']); } }
5. Running the Worker Server
Starting the Worker
You can start processing jobs using the Artisan command:
php artisan queue:work
This command starts a worker that listens to the queue and processes jobs.
Worker Options
- Daemon Mode: Run the worker as a daemon for continuous processing.
php artisan queue:work --daemon
- Specifying Connection and Queue:
php artisan queue:work redis --queue=default
Daemonizing the Worker
In a production environment, you should run the worker as a daemon process. However, running the worker in daemon mode requires careful memory management and regular restarts to prevent memory leaks.
6. Supervising the Worker Process
To ensure your worker processes stay alive, especially after server reboots or crashes, use a process supervisor like Supervisor.
Installing Supervisor
Install Supervisor on Ubuntu:
sudo apt install supervisor
Configuring Supervisor for Laravel
Create a Supervisor configuration file for your Laravel worker:
sudo nano /etc/supervisor/conf.d/laravel-worker.conf
Add the following configuration:
[program:laravel-worker] process_name=%(program_name)s_%(process_num)02d command=php /path/to/your/laravel/artisan queue:work redis --sleep=3 --tries=3 autostart=true autorestart=true user=www-data numprocs=1 redirect_stderr=true stdout_logfile=/path/to/your/laravel/storage/logs/worker.log
Replace /path/to/your/laravel
with the actual path to your Laravel application.
Reloading Supervisor
Update Supervisor to recognize the new configuration:
sudo supervisorctl reread sudo supervisorctl update
Start the worker:
sudo supervisorctl start laravel-worker:*
7. Managing and Monitoring Workers
Handling Failed Jobs
Sometimes, jobs may fail due to exceptions. Laravel provides mechanisms to handle failed jobs.
Setting Up Failed Job Table
Create a migration for the failed jobs table:
php artisan queue:failed-table php artisan migrate
Configuring Retry Mechanisms
You can configure the number of times a job should be retried in the config/queue.php
file or by using the --tries
option when starting the worker.
Monitoring Worker Performance
Monitoring is crucial for maintaining the health of your worker processes.
Using Laravel Horizon (Optional)
For a more advanced monitoring solution, consider using Laravel Horizon, which provides a dashboard to monitor your queues (requires Redis).
Install Horizon:
composer require laravel/horizon
Publish the configuration and assets:
php artisan horizon:install
Run migrations:
php artisan migrate
Update your Supervisor configuration to run Horizon instead of the default worker.
8. Scaling Workers
Running Multiple Workers
To handle a larger volume of jobs, you can increase the numprocs
value in your Supervisor configuration:
numprocs=5
This will start five worker processes.
Optimizing Worker Performance
- Optimize Your Jobs: Ensure that your job logic is efficient.
- Use Cache Wisely: Leverage caching to reduce database load.
- Adjust Worker Sleep Time: Modify the
--sleep
option to control how long the worker waits when no jobs are available.
9. Troubleshooting Common Issues
Debugging Workers
- Check Logs: Review
storage/logs/laravel.log
and the worker logs defined in your Supervisor configuration. - View Failed Jobs: Use
php artisan queue:failed
to list failed jobs. - Retry Failed Jobs: Use
php artisan queue:retry all
to retry all failed jobs.
Common Errors
- Connection Refused: Ensure Redis is running and the connection details are correct.
- Permission Denied: Verify that the user specified in Supervisor has the necessary permissions.
Best Practices
- Secure Your Queue: If using a remote queue service, secure the connection.
- Regularly Update Dependencies: Keep your Laravel application and dependencies up to date.
- Monitor Resource Usage: Keep an eye on CPU and memory usage to prevent overload.
By setting up Laravel workers on Ubuntu, you've taken a significant step toward optimizing your application's performance and scalability. Offloading tasks to background processes not only improves user experience but also allows your application to handle more concurrent requests efficiently.
Next Steps
- Implement More Jobs: Identify additional tasks that can be offloaded.
- Explore Laravel Horizon: For advanced monitoring and management.
- Scale Horizontally: Consider adding more servers as your application grows.
Additional Resources
By following this guide, you're well on your way to mastering Laravel workers and building robust, scalable applications. Happy coding!